Note
Go to the end to download the full example code.
Single bead evaluation plot#
This example shows how to use PyAdditive-Widgets to visualize the results
of a parametric study containing single bead simulations.
It uses the display
package to
visualize the results of the study.
Units are SI (m, kg, s, K) unless otherwise noted.
Perform required imports and create study#
Perform the required imports and create a ParametricStudy
instance.
from ansys.additive.core import Additive, SimulationStatus, SimulationType
from ansys.additive.core.parametric_study import ColumnNames, ParametricStudy
from ansys.additive.widgets import display
study = ParametricStudy("single-bead-study")
Saving parametric study to /home/runner/work/pyadditive-widgets/pyadditive-widgets/examples/single-bead-study.ps
Get name of study file#
The current state of the parametric study is saved to a file on each update. The following code retrieves the name of the file. This file uses a binary format and is not human readable.
print(study.file_name)
/home/runner/work/pyadditive-widgets/pyadditive-widgets/examples/single-bead-study.ps
Select material for study#
Select a material to use in the study. The material name must be known by the Additive service. You can connect to the Additive service and print a list of available materials prior to selecting one.
additive = Additive()
additive.materials_list()
material = "IN718"
2024-06-14 17:22:20 Connected to dns:///localhost:50052
user data path: /home/runner/.local/share/pyadditive
Create single bead evaluation#
Generate single bead simulation permutations using the
generate_single_bead_permutations()
method. This method’s
parameters allow you to specify a range of machine parameters and filter them by
energy density. Not all the parameters shown are required. Optional parameters
that are not specified use the default values defined in the
MachineConstants
class.
import numpy as np
# Specify a range of laser powers. Valid values are 50 to 700 W.
initial_powers = np.linspace(50, 700, 7)
# Specify a range of laser scan speeds. Valid values are 0.35 to 2.5 m/s.
initial_scan_speeds = np.linspace(0.35, 2.5, 5)
# Specify powder layer thicknesses. Valid values are 10e-6 to 100e-6 m.
initial_layer_thicknesses = [40e-6, 50e-6]
# Specify laser beam diameters. Valid values are 20e-6 to 140e-6 m.
initial_beam_diameters = [80e-6]
# Specify heater temperatures. Valid values are 20 - 500 C.
initial_heater_temps = [80]
# Restrict the permutations within a range of energy densities
# For a single bead, the energy density is laser power / (laser scan speed * layer thickness).
min_energy_density = 2e6
max_energy_density = 8e6
# Specify a bead length in meters.
bead_length = 0.001
study.generate_single_bead_permutations(
material_name=material,
bead_length=bead_length,
laser_powers=initial_powers,
scan_speeds=initial_scan_speeds,
layer_thicknesses=initial_layer_thicknesses,
beam_diameters=initial_beam_diameters,
heater_temperatures=initial_heater_temps,
min_area_energy_density=min_energy_density,
max_area_energy_density=max_energy_density,
)
Show simulations as a table#
Use the display
package to list the simulations as a table.
display.show_table(study)
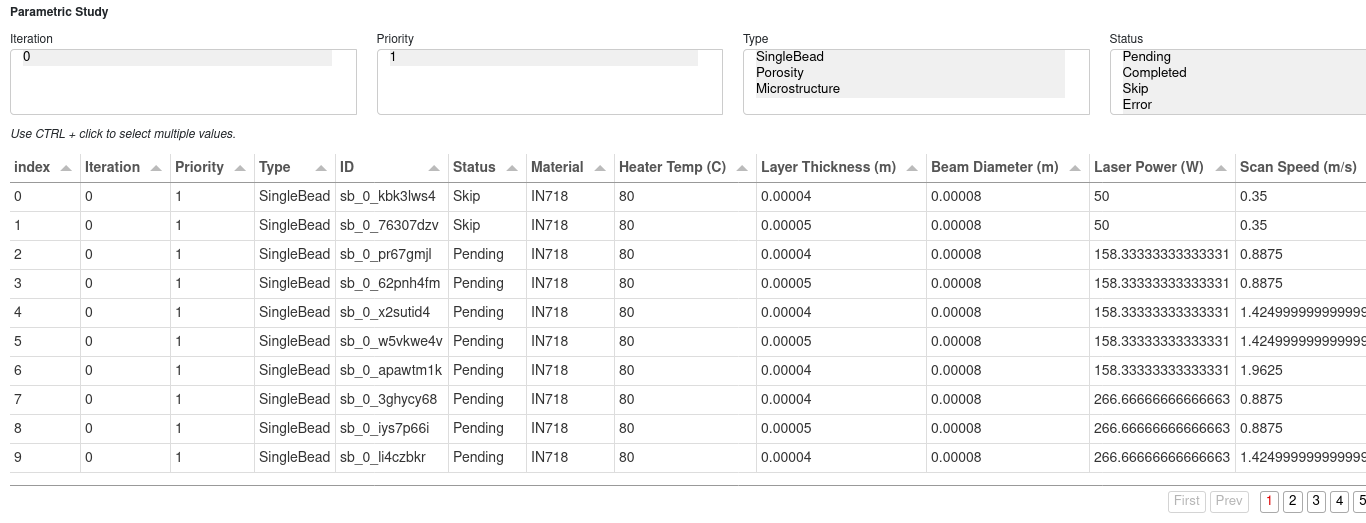
show_table
Skip some simulations#
If you are working with a large parametric study, you might want to skip some
simulations to reduce processing time. To do so, set the simulation status,
which is defined in the SimulationStatus
class, to SimulationStatus.SKIP
.
The following code obtains a DataFrame
object, applies a filter to
get a list of simulation IDs, and then updates the status on the simulations
with those IDs.
df = study.data_frame()
# Get IDs for single bead simulations with laser power below 75 W.
ids = df.loc[
(df[ColumnNames.LASER_POWER] < 75) & (df[ColumnNames.TYPE] == SimulationType.SINGLE_BEAD),
ColumnNames.ID,
].tolist()
study.set_status(ids, SimulationStatus.SKIP)
display.show_table(study)
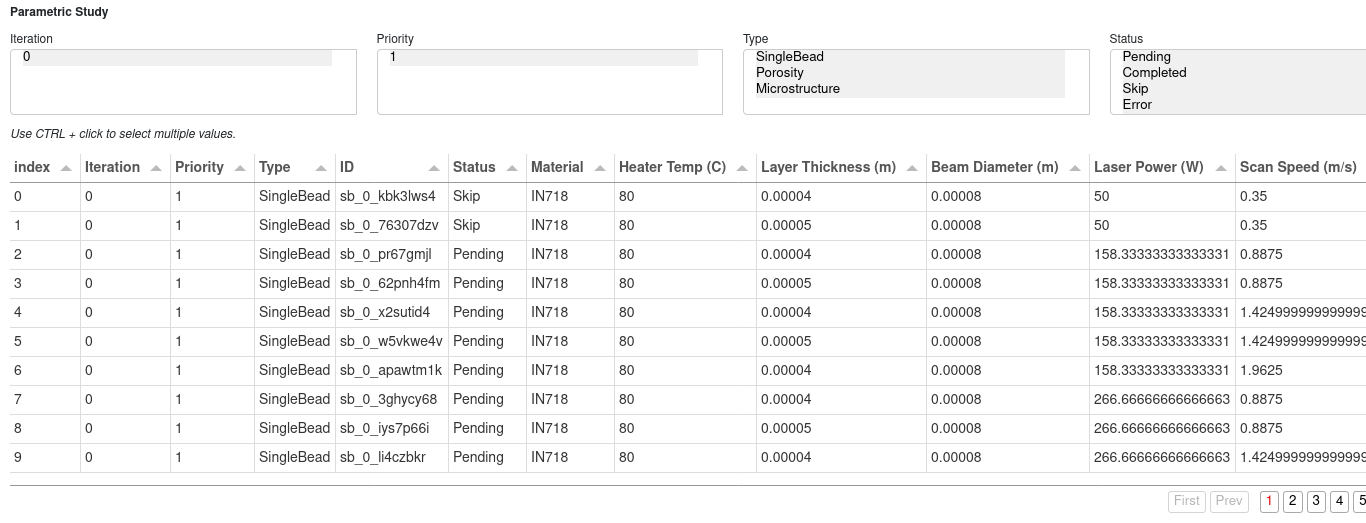
show_table
Run single bead simulations#
Run the simulations using the run_simulations()
method.
All simulations with a SimulationStatus.PENDING
status are executed.
study.run_simulations(additive)
2024-06-14 17:33:11 Completed 0 of 62 simulations
2024-06-14 17:33:33 Completed 1 of 62 simulations
2024-06-14 17:33:50 Completed 2 of 62 simulations
2024-06-14 17:34:07 Completed 3 of 62 simulations
2024-06-14 17:34:21 Completed 4 of 62 simulations
2024-06-14 17:34:43 Completed 5 of 62 simulations
2024-06-14 17:35:07 Completed 6 of 62 simulations
2024-06-14 17:35:23 Completed 7 of 62 simulations
2024-06-14 17:35:42 Completed 8 of 62 simulations
2024-06-14 17:35:56 Completed 9 of 62 simulations
2024-06-14 17:36:10 Completed 10 of 62 simulations
2024-06-14 17:36:23 Completed 11 of 62 simulations
2024-06-14 17:36:37 Completed 12 of 62 simulations
2024-06-14 17:36:53 Completed 13 of 62 simulations
2024-06-14 17:37:10 Completed 14 of 62 simulations
2024-06-14 17:37:24 Completed 15 of 62 simulations
2024-06-14 17:37:38 Completed 16 of 62 simulations
2024-06-14 17:37:51 Completed 17 of 62 simulations
2024-06-14 17:38:05 Completed 18 of 62 simulations
2024-06-14 17:38:23 Completed 19 of 62 simulations
2024-06-14 17:38:37 Completed 20 of 62 simulations
2024-06-14 17:38:52 Completed 21 of 62 simulations
2024-06-14 17:39:04 Completed 22 of 62 simulations
2024-06-14 17:39:19 Completed 23 of 62 simulations
2024-06-14 17:39:33 Completed 24 of 62 simulations
2024-06-14 17:39:48 Completed 25 of 62 simulations
2024-06-14 17:40:00 Completed 26 of 62 simulations
2024-06-14 17:40:15 Completed 27 of 62 simulations
2024-06-14 17:40:30 Completed 28 of 62 simulations
2024-06-14 17:40:54 Completed 29 of 62 simulations
2024-06-14 17:41:16 Completed 30 of 62 simulations
2024-06-14 17:41:29 Completed 31 of 62 simulations
2024-06-14 17:41:42 Completed 32 of 62 simulations
2024-06-14 17:42:06 Completed 33 of 62 simulations
2024-06-14 17:42:22 Completed 34 of 62 simulations
2024-06-14 17:42:39 Completed 35 of 62 simulations
2024-06-14 17:42:53 Completed 36 of 62 simulations
2024-06-14 17:43:15 Completed 37 of 62 simulations
2024-06-14 17:43:40 Completed 38 of 62 simulations
2024-06-14 17:43:56 Completed 39 of 62 simulations
2024-06-14 17:44:13 Completed 40 of 62 simulations
2024-06-14 17:44:27 Completed 41 of 62 simulations
2024-06-14 17:44:42 Completed 42 of 62 simulations
2024-06-14 17:44:54 Completed 43 of 62 simulations
2024-06-14 17:45:09 Completed 44 of 62 simulations
2024-06-14 17:45:25 Completed 45 of 62 simulations
2024-06-14 17:45:38 Completed 46 of 62 simulations
2024-06-14 17:45:56 Completed 47 of 62 simulations
2024-06-14 17:46:11 Completed 48 of 62 simulations
2024-06-14 17:46:24 Completed 49 of 62 simulations
2024-06-14 17:46:38 Completed 50 of 62 simulations
2024-06-14 17:46:56 Completed 51 of 62 simulations
2024-06-14 17:47:10 Completed 52 of 62 simulations
2024-06-14 17:47:25 Completed 53 of 62 simulations
2024-06-14 17:47:38 Completed 54 of 62 simulations
2024-06-14 17:47:52 Completed 55 of 62 simulations
2024-06-14 17:48:06 Completed 56 of 62 simulations
2024-06-14 17:48:21 Completed 57 of 62 simulations
2024-06-14 17:48:34 Completed 58 of 62 simulations
2024-06-14 17:48:48 Completed 59 of 62 simulations
2024-06-14 17:49:04 Completed 60 of 62 simulations
2024-06-14 17:49:18 Completed 61 of 62 simulations
2024-06-14 17:49:31 Completed 62 of 62 simulations
Plot single bead results#
Plot the single bead results using the
single_bead_eval_plot()
function.
display.single_bead_eval_plot(study)
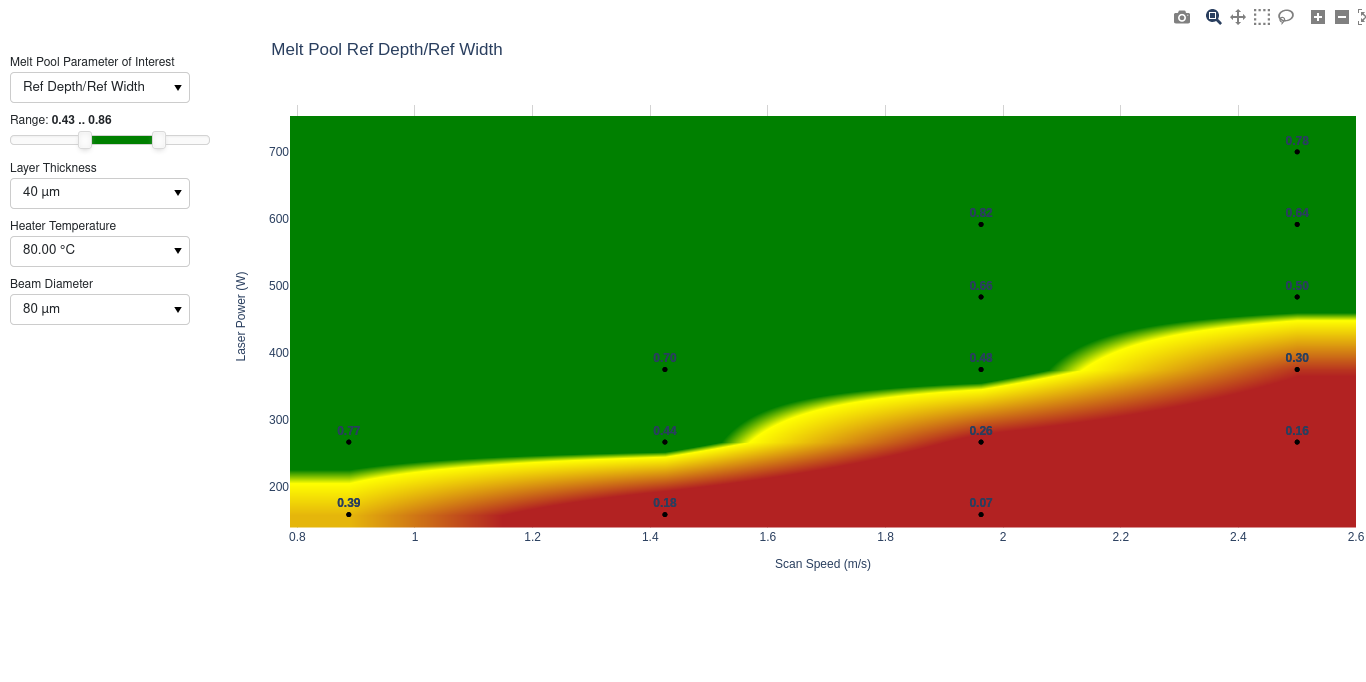
single_bead_eval_plot
Total running time of the script: (27 minutes 15.449 seconds)